JavaScript Fundamentals
An introduction of JS and the DOM
April 30, 2022 by Tim Greenslade
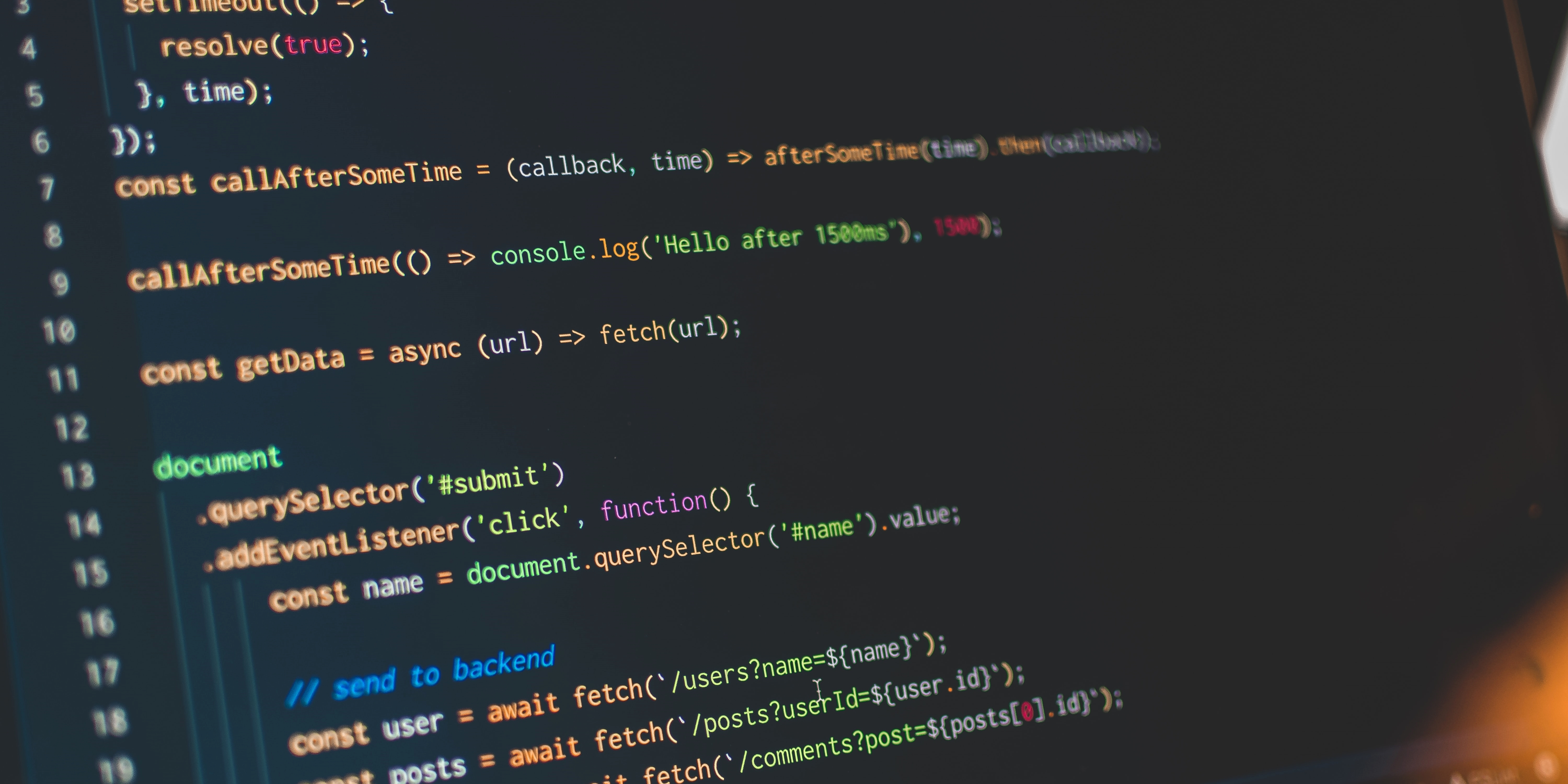
Lets Begin with An analogy to describe how JavaScript relates to HTML and CSS:
Lets say a film has three key elements:
- the script - lays out the bones of the film, like HTML.
- the sets - renders the film visually, like CSS.
- the performers - bring the film to life, like JS.
You could have a film with scripts and sets, but it would be dull. It takes performers to bring a film to life. like JavaScript, they create interactivity and allows events to occur.
An Everyday Example of Control Flow and Loops
Control flow and loops can be used to search through information, and select items, and fulfill functions, based on sets of conditions.
An everyday example of this can be experienced in the chips section at your local supermarket:
The following story is based on true events that occurred in Auckland, New Zealand
I like chips. I'm a chip connoisseur with particular tastes.
I visited a supermarket to look for some thick, wrinkled bbq chips.
I scanned the row of chips, asking each item the following set of questions:
- "Are you thick?"
- "Are you wrinkly?"
- "Are you bbq flavoured?"
After browsing row after row, shelf after shelf, my investigation rendered a list of chips that strictly met my requirements.
I purchased them and went home.
What is the DOM?
The Document Object Model(DOM)'s role is to represent and provide a means to manipulate the Document (or webpage). It acts as an interface for web documents and the structure they create. The DOM represents this structure like a tree, with branches ending in nodes and objects.
An example of a branch is the connection between the Document and an .html document. An example of a node can be found within this .html document, which is in fact comprised entirely by a collection of nodes, some examples would be a header, a main, a div and text elements.
How can we manipulate the DOM?
On a functional level, the DOM takes HTML and CSS and transforms them into Javascript, which can then be manipulated. Effectively, we are able to manipulate everything in the document with Javascript.
Some examples:
Select all instances of a specific element:
document.querySelectorAll('p')
Enter information into an element
getElementById('title').innerHTML = 'This is the worlds greatest title'
Accessing data from Arrays and Objects.
Arrays and Object store collections of information. The ways in which we access data from them are very different. Lets explore:
Accessing data from Arrays:
When an Array stores an item, that item is assigned an index number. That index number is then used to identify specific items in an Array.
What an Array looks like:
let favGhibliFilms = ['Only Yesterday', 'Whisper of the Heart', 'My Neighbor Totoro', 'Laputa']
Here are some ways to interact with an Array:
To access an item in the Array:
console.log(favGhibliFilms[0])
would select 'Only Yesterday'
console.log(favGhibliFilms[3])
would select 'Laputa'To replace an item in the Array:
favGhibliFilms[3] = 'Ponyo'
would change 'Laputa' to 'Ponyo'.To add a new item to the Array:
favGhibliFilms.push('Spirited Away')
would add 'Spirited Away'
Accessing data from Objects:
An Object is used to store information in lists of Key-Value Pairs
Each item is stored with a user assigned keyword, such as 'name', 'numOfPets', 'address' and so on.
What an Object Looks like:
let tim = {
name: 'tim',
age: 33,
eyeColor: 'hazel',
plants: ['Chives', 'Basil'],
partner: 'chloe',
}
Here are some ways to interact with an Object:
To access an item in an Object:
console.log(tim.age)
would print 33.To add an item to an Object
tim.favCountry = 'japan';
To delete a key-value pair:
delete tim.plants;
Objects can be accessed with two different syntaxes:
Square Bracket syntax
tim['age]
It can be used with variables to access information.
Dot syntax
tim.age
It is limited to referencing keys in the Object directly
To illustrate the difference:
let tim = {
name: 'tim',
age: 33,
isCool: true,
eyeColor: 'hazel',
plants: ['Chives', 'Basil'],
}
let key = 'age'
console.log(tim[key])
console.log(tim.key)
Outcome:
console.log(tim[key])
returns "33" because it is searching the Object via a function
console.log(tim.key)
returns "undefined" because it is searching the Object for a Key named "key".
Functions and their use
Functions allow our code to perform tasks, they contain a set of instructions and conditions necessary to complete those tasks.
These tasks are entirely defined by us and are near infinitely customisable. They essentially unlock the world of JavaScript.